This post demonstrates how to develop your own Text to Speech application simply a "Jarvis".
This application is written to just show how simple it is to write text to speech in C#. This application is not fully developed (no multi-threading, no asynchronous calls).
Please follow the blog for fully functional Text to Speech application.
This is how our Jarvis looks like -
Follow these steps to create your own Jarvis -
1. Create a new project of type "Windows Forms Application" targeting .NET Framework 4
Refer to this tutorial How to add a reference to a project
2. Add a reference to System.Speech library
3. Add a System.Speech.Synthesis namespace to your application
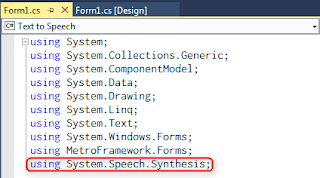
4. Modify Form1.cs code as shown below
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Windows.Forms; using MetroFramework.Forms; using System.Speech.Synthesis; namespace Text_to_Speech { public partial class Form1 : MetroForm { private static SpeechSynthesizer synth = new SpeechSynthesizer(); public Form1() { InitializeComponent(); } private void btnRead_Click(object sender, EventArgs e) { VoiceGender voiceGender = VoiceGender.NotSet; int rate = trackBarRate.Value; //assign voice Gender based on selection if(rdMale.Checked) { voiceGender = VoiceGender.Male; } else { voiceGender = VoiceGender.Female; } //set speech rate synth.Rate = rate; //set voice gender from user selection synth.SelectVoiceByHints(voiceGender); //welcome message synth.Speak("Welcome to Jarvis Application, Version one point oh!"); //start speech synth.Speak(txtReadText.Text); } } }
5. Copy some text in the text box and click the button Start. So the application starts reading the text in the selected voice type at the given rate.
At the runtime you cannot stop, change voice and rate. To support this we have to add threads in our appication. Please follow this blog for fully developed application.