This post demonstrates how to import CSV or any delimited text file with Unicode encoded in Excel using VBA code.
By default, text files are encoded in ANSI representation on WINDOWS platform.When you try to import the ANSI encoded text files into excel using VBA, you may not see problems with the data appearing in the excel worksheet. But there is a problem when importing a text file other than ANSI encoding.
The Possible Encodings of a Text File –
The following are the available character encoding representations of a text file on WINDOWS platform.
- ANSI (default encoding representation)
- Unicode
- Unicode big endian
- UTF-8
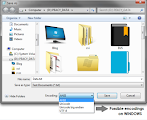
1. Open a text file with notepad editor.
2. Select File menu and click on Save As
3. Look at the Encoding at the bottom, which tells you the current encoding of a text file.
4. Click on Encoding drop-down list, to see the supported encoding representations of a text file on your machine.
What are these encodings?
What happens when Unicode encoded text is imported into Excel –
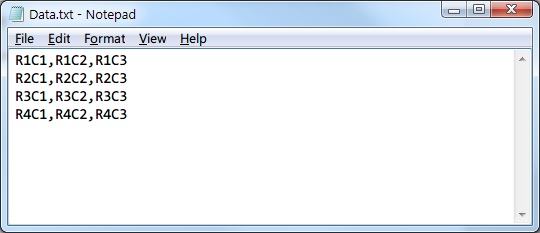
3 Columns
Delimited by ‘,‘ (Comma)
The following is the output screen when each encoded text file is imported into Excel. When the
import
button is clicked, the data will be imported from the active cell in a worksheet.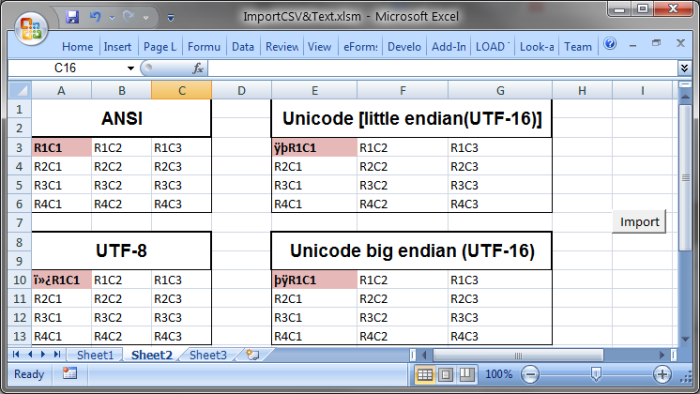
If you look at the output screen, the every first cell of each encoding contains a garbage value.
Reason –
Representation of BOM by encoding –
Encoding | Representation (hexadecimal) | Representation (decimal) | Bytes as CP1252characters |
---|---|---|---|
UTF-8[t 1] | EF BB BF | 239 187 191 |  |
UTF-16(BE) | FE FF | 254 255 | þÿ |
UTF-16(LE) | FF FE | 255 254 | ÿþ |
How to Resolve –
Import Button Code –
1 2 3 | Sub DoTheImport() ImportTextFile FName:="D:\data.txt", Sep:="," End Sub |
ImportFile is the sub-routine which imports the data. Change the sep:= as per your requirement. In this example comma is the separator.ImportFile Code –
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 | Public Sub ImportTextFile(FName As String, Sep As String) Dim RowNdx As Long Dim ColNdx As Integer Dim TempVal As Variant Dim WholeLine As String Dim Pos As Integer Dim NextPos As Integer Dim SaveColNdx As Integer 'disable screen updates Application.ScreenUpdating = False 'error handling On Error GoTo EndMacro 'Importing data starts from the selected cell in a worksheet SaveColNdx = ActiveCell.Column RowNdx = ActiveCell.Row 'open the file in read mode Open FName For Input Access Read As #1 'Read the file until End of Line While Not EOF(1) 'read line by line Line Input #1, WholeLine WholeLine = Replace(WholeLine, "", "", vbTextCompare) 'UTF-8 WholeLine = Replace(WholeLine, "ÿþ", "", vbTextCompare) 'UTF-16 Unicode little endian WholeLine = Replace(WholeLine, "þÿ", "", vbTextCompare) 'UTF-16 Unicode big endian 'checking if the line is empty If Right(WholeLine, 1) <> Sep Then WholeLine = WholeLine & Sep End If ColNdx = SaveColNdx Pos = 1 NextPos = InStr(Pos, WholeLine, Sep) 'finding each column data While NextPos >= 1 TempVal = Mid(WholeLine, Pos, NextPos - Pos) Cells(RowNdx, ColNdx).Value = TempVal Pos = NextPos + 1 ColNdx = ColNdx + 1 NextPos = InStr(Pos, WholeLine, Sep) Wend RowNdx = RowNdx + 1 Wend Close #1 Exit Sub EndMacro: Application.ScreenUpdating = True MsgBox Err.Description, vbOKOnly, "Error" Close #1 End Sub |
Summary –
Please share your thoughts on this post in the comments section.
0 comments:
Post a Comment